Hibernate in Short:
Hibernate is an open source object/relational mapping tool for Java. Hibernate lets you develop persistent classes following common Java idiom - including association, inheritance, polymorphism, composition and the Java collections framework.
Hibernate not only takes care of the mapping from Java classes to database tables (and from Java data types to SQL data types), but also provides data query and retrieval facilities and can significantly reduce development time otherwise spent with manual data handling in SQL and JDBC.
Hibernates goal is to relieve the developer from 95 percent of common data persistence related programming tasks.
Overview:
High level architecture of Hibernate can be described as shown in following illustration.
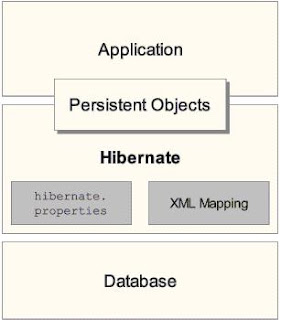
Hibernate makes use of persistent objects commonly called as POJO (POJO = "Plain Old Java Object".) along with XML mapping documents for persisting objects to the database layer. The term POJO refers to a normal Java objects that does not serve any other special role or implement any special interfaces of any of the Java frameworks (EJB, JDBC, DAO, JDO, etc...).
Rather than utilize byte code processing or code generation, Hibernate uses runtime reflection to determine the persistent properties of a class. The objects to be persisted are defined in a mapping document, which serves to describe the persistent fields and associations, as well as any subclasses or proxies of the persistent object. The mapping documents are compiled at application startup time and provide the framework with necessary information for a class. Additionally, they are used in support operations, such as generating the database schema or creating stub Java source files.
Typical Hibernate code
sessionFactory = new Configuration().configure().buildSessionFactory();
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
Customer newCustomer = new Customer();
newCustomer.setName("New Customer");
newCustomer.setAddress("Address of New Customer");
newCustomer.setEmailId("NewCustomer@NewCustomer.com");
session.save(newCustomer);
tx.commit();
session.close();
First step is hibernate application is to retrieve Hibernate Session; Hibernate Session is the main runtime interface between a Java application and Hibernate. SessionFactory allows applications to create hibernate session by reading hibernate configurations file hibernate.cfg.xml.
After specifying transaction boundaries, application can make use of persistent java objects and use session for persisting to the databases.
Features:
Ø Transparent persistence without byte code processing
Ø Object-oriented query language
Ø Object / Relational mappings
Ø Automatic primary key generation
Ø Object/Relational mapping definition
Ø HDLCA (Hibernate Dual-Layer Cache Architecture)
Ø High performance
Ø J2EE integration
We will take up an simple java example that authenticates users based on the credentials to get started with hibernate.
Preparing Database
Let’s consider a simple database schema with a singe table as APPLABSUSER.
CREATE TABLE `applabsuser` (
`USER_ID` int(11) NOT NULL default '0',
`USER_NAME` varchar(25) NOT NULL default '',
`USER_PASSWORD` varchar(25) NOT NULL default '',
`USER_FIRST_NAME` varchar(25) NULL default,
`USER_LAST_NAME` varchar(25) NULL default,
`USER_EMAIL` varchar(25) NULL default,
`USER_CREATION_DATE` date NULL default,
`USER_MODIFICATION_DATE` date NULL default,
PRIMARY KEY (`USER_ID`),
UNIQUE KEY `USER_NAME` (`USER_NAME`)
) ;
Creating persistent java objects
Hibernate works best with the Plain Old Java Objects programming model for persistent classes.
Hibernate is not restricted in its usage of property types, all Java JDK types and primitives (like String, char and Date) can be mapped, including classes from the Java collections framework. You can map them as values, collections of values, or associations to other entities. The id is a special property that represents the database identifer (primary key) of that class, Hibernate can use identifiers only internally, but we would lose some of the flexibility in our application architecture.
No special interface has to be implemented for persistent classes nor do you have to subclass from a special root persistent class. Hibernate also doesn't require any build time processing, such as byte-code manipulation, it relies solely on Java reflection and runtime class enhancement (through CGLIB). So, without any dependency of the POJO class on Hibernate, we can map it to a database table.
Following code sample represents a java object structure which represents the AppLabsUser table. Generally these domain objects contain only getters and setters methods. One can use Hibernate extension toolset to create such domain objects.
AppLabsUser.java
import java.io.Serializable;
import java.util.Date;
import org.apache.commons.lang.builder.ToStringBuilder;
public class AppLabsUser implements Serializable {
public void setName(String name) {
private Long id;/** identifier field */
pivate String userName;
private String userPassword;
private String userFirstName;
private String userLastName;
private String userEmail;
private Date userCreationDate;
private Date userModificationDate;
/** full constructor */
public Applabsuser(String userName, String userPassword, String userFirstName, String userLastName, String userEmail, Date userCreationDate, Date userModificationDate) {
this.userName = userName;
this.userPassword = userPassword;
this.userFirstName = userFirstName;
this.userLastName = userLastName;
this.userEmail = userEmail;
this.userCreationDate = userCreationDate;
this.userModificationDate = userModificationDate;
}
/** default constructor */
public Applabsuser() {}
public Long getId() {return this.id;}
public void setId(Long id) {this.id = id;}
public String getUserName() {return this.userName;}
public void setUserName(String userName) {
this.userName = userName;}
public String getUserPassword() {return this.userPassword;}
public void setUserPassword(String userPassword) {
this.userPassword = userPassword;}
public String getUserFirstName() {return this.userFirstName;}
public void setUserFirstName(String userFirstName) {
this.userFirstName = userFirstName;}
public String getUserLastName() {return this.userLastName;}
public void setUserLastName(String userLastName) {this.userLastName = userLastName;}
public String getUserEmail() {return this.userEmail;}
public void setUserEmail(String userEmail) {this.userEmail = userEmail;}
public Date getUserCreationDate() {return this.userCreationDate;}
public void setUserCreationDate(Date userCreationDate) {
this.userCreationDate = userCreationDate;}
public Date getUserModificationDate() {
return this.userModificationDate;}
public void setUserModificationDate(Date userModificationDate) {
this.userModificationDate = userModificationDate;}
return new ToStringBuilder(this).append("id", getId()).toString();}
} // End of class
Mapping POJO with persistence layer using hibernate mapping document
Each persistent class needs to be mapped with its configuration file. Following code represents Hibernate mapping file for AppLabsUser class.
One can also generate Hibernate mapping documents using Hibernate extension toolset. Hibernate mapping documents are straight forward. TheHibernate Configuration File
Hibernate configuration file information needed to connect to persistent layer and the linked mapping documents. You can either specify the data source name or JDBC details that are required for hibernate to make JDBC connection to the database. The element
| ||
| | |
| | |
| | |
|
Hibernate Sample Code (Inserting new record)
Here is how you can use Hibernate in your programs. Typical Hibernate programs begin with configuration that is required for Hibernate. Hibernate can be configured in two ways. Programmatically and Configuration file based. In Configuration file based mode, hibernate looks for configuration file “hibernate.cfg.xml” in the claspath. Based on the resource mapping provided hibernate creates mapping of tables and domain objects. In the programmatic configuration method, the details such as JDBC connection details and resource mapping details etc are supplied in the program using Configuration API.
Following example shows programmatic configuration of hibernate.
| |
Configuration config = new Configuration() |
In configuration file based approach, “hibernate.cfg.xml” is placed in the classpath, Following Hibernate code can be used in this method.
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory(); |
Hibernate Sample Code (Querying the database)
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory(); Session session = sessionFactory.openSession(); ArrayList arrayList = null; String SQL_STRING = "FROM AppLabsUser as users"; Query query = session.createQuery(SQL_STRING); ArrayList list = (ArrayList)query.list(); for(int i=0; i System.out.println(list.get(i)); } session.close(); |
Hibernate O/R Mapping
Object/relational mappings are usually defined in XML document. The mapping language is Java-centric, meaning that mappings are constructed around persistent class declarations, not table declarations.
AppLabsUser.hbm.xml
No comments:
Post a Comment